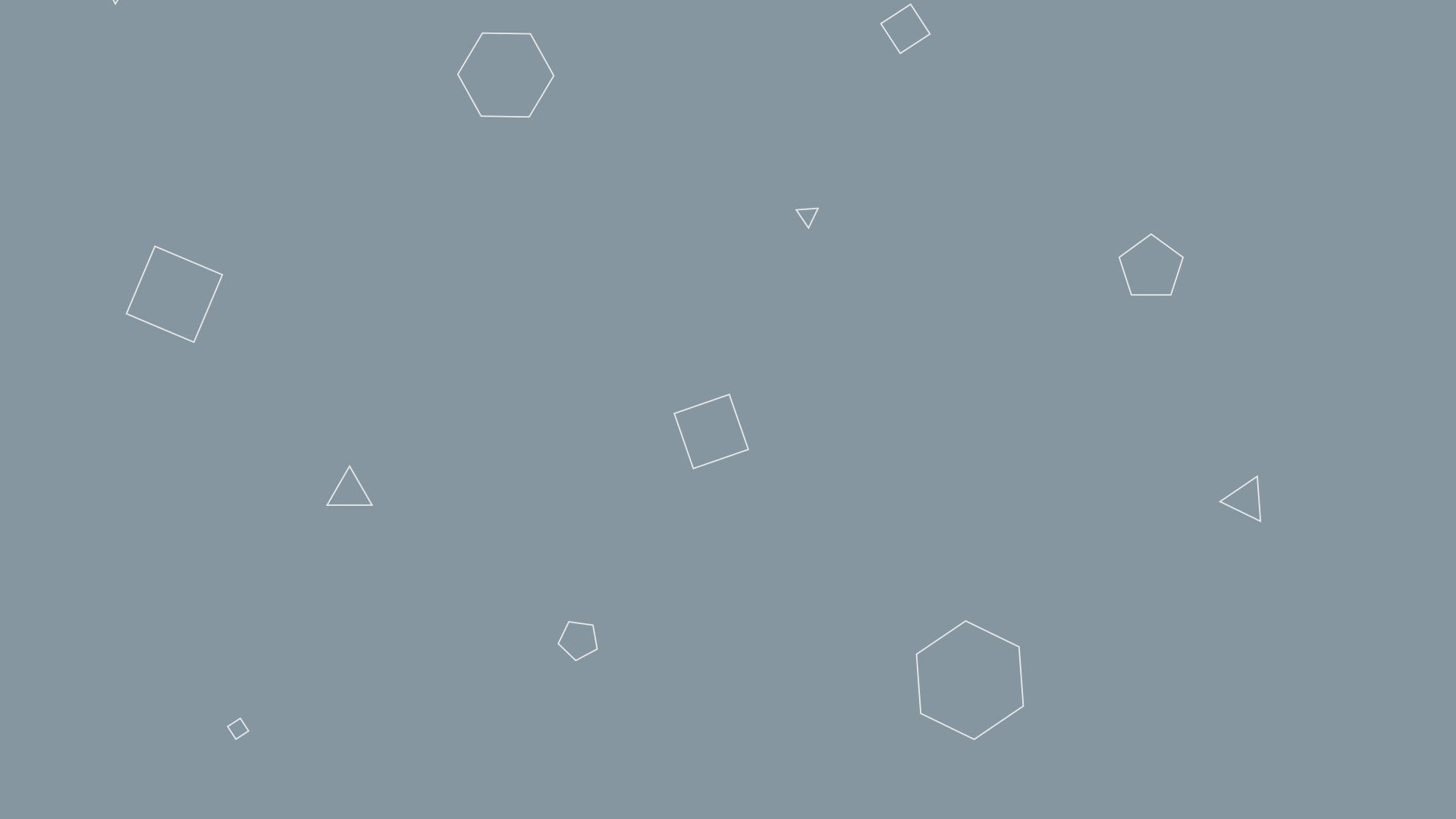
C++ Christmas Tree
#include <iostream>
#include <ctime>
#include <windows.h>
#include <iomanip>
#pragma warning(disable : 4996)
using namespace std;
HANDLE color = GetStdHandle(STD_OUTPUT_HANDLE);
const char BLANK = ' '; //constant: symbol used for drawing the space around the tree
const char LEAF = '#'; //constant: symbol used for drawing the tree’ leaves’
const char WOOD = '|'; //constant: symbol used for drawing the tree’s trunk
const char border = '.';
int main()
{
void drawAXmasTree();
void displayTime();
cout << "Name: Daniel Lomax - Tutorial Group: 2 - Deadline: 12/12/2017\n";
displayTime();
drawAXmasTree();
system("pause");
return (0);
}
void drawAXmasTree()
{
int getValidHeight();
void drawFoliage(int, int&);
void drawTrunk(int);
void agreeToContinue();
int branchLine=1;
int treeHeight = getValidHeight();
drawFoliage(treeHeight, branchLine); //draw tree foliage
drawTrunk(treeHeight); //draw tree trunk
agreeToContinue();
}
int getValidHeight()
{
const int MINSIZE = 4; //constant: symbol used for checking min tree height
const int MAXSIZE = 20; //constant: symbol used for checking max tree height
int treeHeight;
cout << "Enter the size of the tree (4-20): ";
cin >> treeHeight;
while ((treeHeight < MINSIZE) || (treeHeight > MAXSIZE))
{
cout << "\nERROR: Invalid height! Enter the size of the tree (4-20): ";
cin >> treeHeight;
}
return (treeHeight);
}
void drawFoliage(int treeHeight, int& branchLine) //draw the foliage of the tree
{
void drawALineOfFoliage(int, int&);
do
{
drawALineOfFoliage(treeHeight, branchLine); //TO BE DEFINED
branchLine = branchLine + 1;
} while (branchLine <= (treeHeight - 2));
}
void drawTrunk(int treeHeight) //draw the trunk of the tree
{
int trunkLine, spaces;
int row = treeHeight - 2; // change row to branch
int i = 1;
trunkLine = 1;
SetConsoleTextAttribute(color, 8);
do // for each line in the truck
{
SetConsoleTextAttribute(color, 15);
cout << ".";
spaces = 1;
do //draw the spaces on the left
{
cout << BLANK;
spaces = spaces + 1;
} while (spaces <= (treeHeight - 3));
cout << WOOD; //draw the truck
for (int spaces = 1; spaces <= row - i; ++spaces)
{
cout << BLANK;
}
cout << ".";
cout << "\n"; //go to next line
trunkLine = trunkLine + 1;
} while (trunkLine <= 2);
for (int length = 0; length <= treeHeight-3; length++)
{
SetConsoleTextAttribute(color, 15);
cout << ".-";
}
cout << "\n";
}
void drawALineOfFoliage(int treeHeight, int& branchLine)
{
void randomDecoration();
for (int length = 0; length <= treeHeight - 3; length++)
{
SetConsoleTextAttribute(color, 15);
cout << ".-";
}
cout << "\n";
int row = treeHeight - 2; // change row to branch
int i = 1;
int k = 0;
for (i, k; i <= row; ++i, k = 0)
{
SetConsoleTextAttribute(color, 15);
cout << ".";
branchLine = i;
for (int space = 1; space <= row - i; ++space)
{
cout << BLANK;
}
if (i == 1)
{
randomDecoration();
k++;
}
while (k != ((2 * i) - 1))
{
if (i != 1)
{
SetConsoleTextAttribute(color, 2);
cout << LEAF;
++k;
}
else
{
++k;
}
}
for (int space = 1; space <= row - i; ++space)
{
cout << BLANK;
}
SetConsoleTextAttribute(color, 15);
cout << ".";
cout << "\n";
}
}
void randomDecoration()
{
int dec;
srand(time(0));
dec = (rand() % 4);
SetConsoleTextAttribute(color, 6);
switch (dec)
{
case 0: cout << "*";
break;
case 1: cout << "&";
break;
case 2: cout << "@";
break;
case 3: cout << "0";
break;
}
}
void agreeToContinue()
{
char more;
cout << "would you like another tree? (Y/N): ";
cin >> more;
if (more = (toupper('y')))
{
drawAXmasTree();
}
}
void displayTime()
{
time_t t = time(0); // get time now
struct tm * now = localtime(&t);
int month = (now->tm_mon + 1);
cout << "Date: "
<< (now->tm_year + 1900) << "-";
switch (month)
{
case 1: cout << "January - ";
case 2:cout << "February - ";
case 3:cout << "March - ";
case 4:cout << "April - ";
case 5:cout << "May - ";
case 6:cout << "June - ";
case 7:cout << "July - ";
case 8:cout << "August - ";
case 9:cout << "September - ";
case 10:cout << "October - ";
case 11:cout << "November - ";
case 12:cout << "December - ";
}
cout << now->tm_mday << " "
<< "Time: "
<< now->tm_hour << ":"
<< now->tm_min << ":"
<< now->tm_sec
<< "\n";
}
