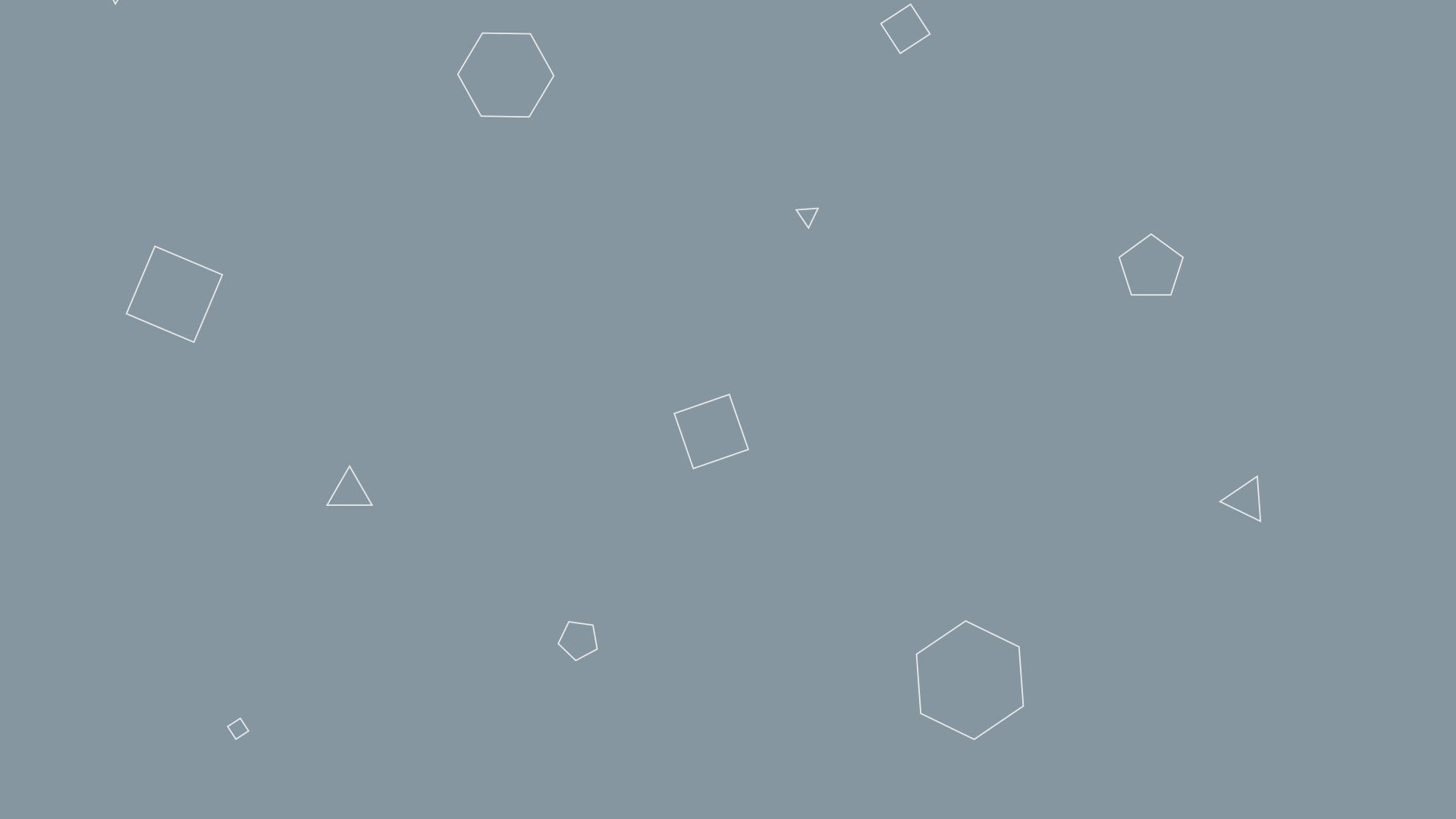
C++ Zombie game
//---------------------------------------------------------------------------
//Program: Skeleton for Task 1c – group assignment
//---------------------------------------------------------------------------
//---------------------------------------------------------------------------
//----- include libraries
//---------------------------------------------------------------------------
//include standard libraries
#include <iostream>
#include <iomanip>
#include <conio.h>
#include <cassert>
#include <string>
#include <sstream>
#include <vector>
#include <fstream>
using namespace std;
//include our own libraries
#include "RandomUtils.h" //for Seed, Random
#include "ConsoleUtils.h" //for Clrscr, Gotoxy, etc.
#include "TimeUtils.h"
//---------------------------------------------------------------------------
//----- define constants
//---------------------------------------------------------------------------
//defining the size of the grid
const int SIZEX(25); //horizontal dimension
const int SIZEY(15); //vertical dimension
//defining symbols used for display of the grid and content
const char SPOT('@'); //spot symbol
const char TUNNEL(' '); //tunnel symbol
const char HOLE('0'); //hole symbol
const char ZOMBIE('Z'); //zombie symbol
const char PILL('*'); //pill symbol
const char WALL('#'); //border symbol
//defining the command letters to move the spot on the maze
const int UP(72); //up arrow
const int DOWN(80); //down arrow
const int RIGHT(77); //right arrow
const int LEFT(75); //left arrow
//define cheat keys
const char FREEZE('F'); // freeze zombie positions
const char EAT('E'); // eat all pills
const char EXTERMINATE('X');// kill all zombies
bool Legit = true; //a toggle to see if the player has cheated
const int ZombieLocations[4][2] = //zombie spawnpoints
{
{ 2,2 },
{ SIZEX - 1,2 },
{ 2, SIZEY - 2 },
{ SIZEX - 2, SIZEY - 2 }
};
//defining the other command letters
const char QUIT('Q'); //to end the game
struct Item //define the Items components
{
int x, y; // position
char symbol; // identifier
};
//---------------------------------------------------------------------------
//----- run game
//---------------------------------------------------------------------------
int main()
{
//function declarations (prototypes)
void Death(const char g[][SIZEX], Item spot, int& Lives, int& key);
void paintEntryScreen(bool cP, string& name);
void initialiseGame(char g[][SIZEX], char m[][SIZEX], Item& spot, vector<Item>& hole, vector<Item>& zombies, vector<Item>& pills, bool& exterminate, int MAXZOMBIES, bool& freezeActive, int Score, int& Lives, int& zombiesLeft, int& key);
void paintGame(const char g[][SIZEX], string mess, int& Lives, int& pillsLeft, int zombiesLeft, string& name);
bool wantsToQuit(const int key);
bool isArrowKey(const int k);
bool isCheatKey(const int k);
int getKeyPress();
void usedCheatKey(const int key, vector<Item>& pills, int MAXPILLS, int& pillsLeft, int zombiesLeft, bool& exterminate, bool& freezeActive, int& Lives);
void updateGameData(char g[][SIZEX], Item& spot, int key, string& mess, int& pillsLeft, int zombiesLeft, int& Lives, vector<Item>& pills);
void updateGrid(char g[][SIZEX], const char m[][SIZEX], const Item spot, vector<Item>& hole, vector<Item>& zombies, vector<Item>& pills, bool& exterminate, int MAXZOMBIES, bool& freezeActive, int Score, int& Lives, int& zombiesLeft, int& key);
void endProgram(int &key);
bool canPaint = false;
char grid[SIZEY][SIZEX]; //grid for display
char maze[SIZEY][SIZEX]; //structure of the maze
Item spot = { 0, 0, SPOT }; //spot's position and symbol
//Hole stuff
const int MAXHOLES(12);
Item hole = { 0, 0, HOLE };
vector<Item> holes(MAXHOLES, hole);
//zombie stuff
const int MAXZOMBIES(4);
Item zombie = { 0, 0, ZOMBIE };
vector<Item> zombies(MAXZOMBIES, zombie);
//pill stuff
const int MAXPILLS(7);
Item pill = { 0, 0, PILL };
vector<Item> pills(MAXPILLS, pill);
int zombiesLeft = 4;
int pillsLeft = 7;
string message("LET'S START..."); //current message to player
//cheat stuff
bool freezeActive = false; // cheat FREEZE - used to stop zombies moving - disables zombieControl
bool exterminate = false; // cheat exterminate - used in zombieContol to destory / create zombies
bool spawnZombies = true; // used for cheat, exterminate
int Lives = 3;
int Score = 0;
string name;
int key; //current key selected by player
//action...
Seed(); //seed the random number generator
SetConsoleTitle("Spot and Zombies Game - FoP 2017-18");
if (!canPaint) // start the game with the title page and enter name
{
paintEntryScreen(canPaint, name);
}
canPaint = true; // trigger play level 1
initialiseGame(grid, maze, spot, holes, zombies, pills, exterminate, MAXZOMBIES, freezeActive, Score, Lives, zombiesLeft, key); //initialise grid (incl. walls and spot)
if (canPaint) // play level 1
{
paintGame(grid, message, Lives, pillsLeft, zombiesLeft, name); //display game info, modified grid and messages
}
do {
key = toupper(getKeyPress()); //read in selected key: arrow or letter command
if (isArrowKey(key)) // move everything and update any changes
{
updateGameData(grid, spot, key, message, pillsLeft,zombiesLeft, Lives , pills); //move spot in that direction
updateGrid(grid, maze, spot, holes, zombies, pills, exterminate, MAXZOMBIES, freezeActive, Score, Lives, zombiesLeft, key); //update grid information
}
else if (isCheatKey(key)) // apply cheat and update the game accordingly
{
usedCheatKey(key, pills, MAXPILLS, pillsLeft, zombiesLeft, exterminate, freezeActive, Lives);
updateGameData(grid, spot, key, message, pillsLeft,zombiesLeft, Lives, pills); //move spot in that direction
updateGrid(grid, maze, spot, holes, zombies, pills, exterminate, MAXZOMBIES, freezeActive, Score, Lives, zombiesLeft, key);
}
else
message = "INVALID KEY!"; //set 'Invalid key' message
paintGame(grid, message, Lives, pillsLeft, zombiesLeft, name); //display game info, modified grid and messages
} while (!wantsToQuit(key)); //while user does not want to quit
endProgram(key); //display final message
return 0;
}
//---------------------------------------------------------------------------
//----- initialise game state
//---------------------------------------------------------------------------
void initialiseGame(char grid[][SIZEX], char maze[][SIZEX], Item& spot, vector<Item>& hole, vector<Item>& zombies, vector<Item>& pills, bool& exterminate, int MAXZOMBIES, bool& freezeActive, int Score, int& Lives, int& zombiesLeft, int& key)
{ //initialise grid and place spot in middle
void setInitialMazeStructure(char maze[][SIZEX]);
void setSpotInitialCoordinates(Item& spot);
void setHoleCoordinates(char g[][SIZEX], vector<Item>& hole);
void updateGrid(char g[][SIZEX], const char m[][SIZEX], Item b, vector<Item>& hole, vector<Item>& zombies, vector<Item>& pills, bool& exterminate, int MAXZOMBIES, bool& freezeActive, int Score, int& Lives, int& zombiesLeft, int& key);
void createZombies(char g[][SIZEX], vector<Item>& zombies);
void setPillCoordinates(char g[][SIZEX], vector<Item>& pills);
setInitialMazeStructure(maze); //initialise maze
updateGrid(grid, maze, spot, hole, zombies, pills, exterminate, MAXZOMBIES, freezeActive, Score, Lives, zombiesLeft, key); //prepare grid
setSpotInitialCoordinates(spot);
createZombies(grid, zombies);
setHoleCoordinates(grid, hole);
setPillCoordinates(grid, pills);
updateGrid(grid, maze, spot, hole, zombies, pills, exterminate, MAXZOMBIES, freezeActive, Score, Lives, zombiesLeft, key); //prepare grid
}
void setSpotInitialCoordinates(Item& spot) // get a random place for the spot it spawn at
{
spot.y = Random(SIZEY - 2); //vertical coordinate in range [1..(SIZEY - 2)]
spot.x = Random(SIZEX - 2); //horizontal coordinate in range [1..(SIZEX - 2)]
}
void setHoleCoordinates(char g[][SIZEX], vector<Item>& holes) // get a random place for each hole to be placed, as long as it's not overlapping anything
{
void placeItem(char g[][SIZEX], const Item& item);
for (size_t i(0); i < holes.size(); ++i) // for every hole
{
int yPos = Random(SIZEY - 2); // random y
int xPos = Random(SIZEX - 2); // random x
while (g[yPos][xPos] != TUNNEL) // if it overlaps anything it shouldn't
{
yPos = Random(SIZEY - 2); // random y
xPos = Random(SIZEX - 2); // random x
}
holes.at(i).y = yPos; // set hole y
holes.at(i).x = xPos; // set hole x
placeItem(g, holes.at(i)); // place hole
}
}
void setPillCoordinates(char g[][SIZEX], vector<Item>& pills) // get a random place for each pill to be placed, as long as it's not overlapping anything
{
void placeItem(char g[][SIZEX], const Item& item);
for (size_t i(0); i < pills.size(); ++i) // for every pill
{
int yPos = Random(SIZEY - 2); // random y
int xPos = Random(SIZEX - 2); // random x
while (g[yPos][xPos] != TUNNEL) // if it overlaps anything it shiuldn't
{
yPos = Random(SIZEY - 2); // random y
xPos = Random(SIZEX - 2); // random x
}
pills.at(i).y = yPos; // set pill y
pills.at(i).x = xPos; // set pill x
placeItem(g, pills.at(i)); // place pill
}
}
void ZombieControl(Item& Zombie, Item spot, char g[][SIZEX], bool& exterminate, int MAXZOMBIES, bool& freezeActive, int Score, int& Lives, int& zombiesLeft, int& key, vector<Item>& zombies, vector<Item>& holes)
{
void createZombies(char g[][SIZEX], vector<Item>& zombies);
void Death(const char g[][SIZEX], Item& spot, int& Lives, int& key);
int randomLocations;
void ScoreIncrease(int Score);
int XDistance;
int yDistance;
int dY(0);
int dX(0);
XDistance = spot.x - Zombie.x; // distance between spot and the zombie
yDistance = spot.y - Zombie.y; //" "
if(XDistance >=30)
{
}
else
{
if (XDistance != 0) // when not on the same x, go towards x
{
if (XDistance > 0)
{
dX = 1;
}
else
{
dX = -1;
}
}
if (yDistance != 0) // when not on the same y, go towards y
{
if (yDistance > 0)
{
dY = 1;
}
else
{
dY = -1;
}
}
}
if (Zombie.x < 50)
{
switch (g[Zombie.y + dY][Zombie.x]) // check what the zombie is moving onto in the y
{
case TUNNEL:
Zombie.y += dY;
break; // can move onto tunnel
case SPOT:
Death(g, spot, Lives, key);
Zombie.y += dY; // kill spot and move onto
randomLocations = Random(4) - 1;
Zombie.x = ZombieLocations[randomLocations][0];
Zombie.y = ZombieLocations[randomLocations][1];
break;
case WALL:
break; // don't move that way
case ZOMBIE: // respawn
randomLocations = Random(4) - 1;
Zombie.x = ZombieLocations[randomLocations][0];
Zombie.y = ZombieLocations[randomLocations][1];
break;
case HOLE: // dies and doesn't come back, increases score
Zombie.y += dY;
ScoreIncrease(Score);
zombiesLeft--;
Zombie.x = 50;
Zombie.y = 50;
Zombie.symbol = ' ';
break;
}
switch (g[Zombie.y][Zombie.x + dX]) // check what the zombie is moving onto in the x
{
case TUNNEL:
Zombie.x += dX;
break; // can move onto tunnel
case SPOT:
Death(g, spot, Lives, key);
Zombie.x += dX;
randomLocations = Random(4) - 1;
Zombie.x = ZombieLocations[randomLocations][0];
Zombie.y = ZombieLocations[randomLocations][1];
break; // kill spot and move onto
case WALL:
break; // don't move that way
case ZOMBIE: // respawn
randomLocations = Random(4) - 1;
Zombie.x = ZombieLocations[randomLocations][0];
Zombie.y = ZombieLocations[randomLocations][1];
break;
case HOLE: // dies and doesn't come back, increases score
Zombie.x += dX;
ScoreIncrease(Score);
zombiesLeft--;
Zombie.x = 50;
Zombie.y = 50;
Zombie.symbol = ' ';
break;
}
}
}
void createZombies(char g[][SIZEX], vector<Item>& zombies)
{
void placeItem(char g[][SIZEX], const Item& spot);
SelectBackColour(clBlack);
SelectTextColour(clGreen);
for (int i = 0; i < 4; i++) // for every zombie
{
zombies.at(i).x = ZombieLocations[i][0]; // place its x spawn position
zombies.at(i).y = ZombieLocations[i][1]; // place its y spawn position
placeItem(g, zombies.at(i)); // place zombie
}
}
void setInitialMazeStructure(char maze[][SIZEX])
{
//initialise maze configuration
char initialMaze[SIZEY][SIZEX] //local array to store the maze structure
= { { '#', '#', '#', '#', '#', '#', '#', '#', '#', '#','#', '#', '#', '#', '#', '#', '#', '#', '#','#', '#' ,'#' ,'#' ,'#', '#' },
{ '#', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ',' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ',' ', ' ', ' ', ' ',' ', '#' },
{ '#', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ',' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ',' ', ' ', ' ', ' ',' ', '#' },
{ '#', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ',' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ',' ', ' ', ' ', ' ',' ', '#' },
{ '#', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ',' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ',' ', ' ', ' ', ' ',' ', '#' },
{ '#', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ',' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ',' ', ' ', ' ', ' ',' ', '#' },
{ '#', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ',' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ',' ', ' ', ' ', ' ',' ', '#' },
{ '#', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ',' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ',' ', ' ', ' ', ' ',' ', '#' },
{ '#', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ',' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ',' ', ' ', ' ', ' ',' ', '#' },
{ '#', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ',' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ',' ', ' ', ' ', ' ',' ', '#' },
{ '#', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ',' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ',' ', ' ', ' ', ' ',' ', '#' },
{ '#', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ',' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ',' ', ' ', ' ', ' ',' ', '#' },
{ '#', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ',' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ',' ', ' ', ' ', ' ',' ', '#' },
{ '#', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ',' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ',' ', ' ', ' ', ' ',' ', '#' },
{ '#', '#', '#', '#', '#', '#', '#', '#', '#','#', '#', '#', '#', '#', '#', '#', '#', '#', '#','#', '#' ,'#' ,'#' ,'#', '#' } };
//with '#' for wall, ' ' for tunnel, etc.
//copy into maze structure with appropriate symbols
for (int row(0); row < SIZEY; ++row)
for (int col(0); col < SIZEX; ++col)
switch (initialMaze[row][col])
{
case '#': maze[row][col] = WALL; break;
case ' ': maze[row][col] = TUNNEL; break;
}
}
//---------------------------------------------------------------------------
//----- update grid state
//---------------------------------------------------------------------------
void updateGrid(char grid[][SIZEX], const char maze[][SIZEX], const Item spot, vector<Item>& holes, vector<Item>& zombies, vector<Item>& pills, bool& exterminate, int MAXZOMBIES, bool& freezeActive, int Score, int& Lives, int& zombiesLeft, int& key)
{ //update grid configuration after each move
void setMaze(char g[][SIZEX], const char b[][SIZEX]);
void placeItem(char g[][SIZEX], const Item& spot);
void placeHoles(char grid[][SIZEX], const vector<Item>& hole);
void placePills(char grid[][SIZEX], const vector<Item>& pills);
setMaze(grid, maze); //reset the empty maze configuration into grid
placeItem(grid, spot); //set spot in grid
placeHoles(grid, holes);
placePills(grid, pills);
for (int i = 0; i < 4; i++) // for every zombie
{
if (exterminate == true) // if exterminate cheat was used
{
zombies.at(i).x = ZombieLocations[i][0]; // set the zombie x position back to its original place
zombies.at(i).y = ZombieLocations[i][1]; // set the zombie y posotion back to its original place
}
else
{
if (freezeActive == true) // if freeze cheat was used
{
placeItem(grid, zombies[i]); // place zombies - not updating thier positions
}
else
{
ZombieControl(zombies[i], spot, grid, exterminate, MAXZOMBIES, freezeActive, Score, Lives, zombiesLeft, key, zombies, holes); //zombies can move
placeItem(grid, zombies[i]); // zombies placed
}
}
}
}
void setMaze(char grid[][SIZEX], const char maze[][SIZEX])
{
//reset the empty/fixed maze configuration into grid
for (int row(0); row < SIZEY; ++row)
for (int col(0); col < SIZEX; ++col)
grid[row][col] = maze[row][col];
}
void placeItem(char g[][SIZEX], const Item& item)
{
//place item at its new position in grid
g[item.y][item.x] = item.symbol;
}
void placeHoles(char grid[][SIZEX], const vector<Item>& holes)
{
//place holes at its new position in grid
void placeItem(char g[][SIZEX], const Item& item);
for (size_t i(0); i < holes.size(); ++i)
placeItem(grid, holes.at(i));
}
void placePills(char grid[][SIZEX], const vector<Item>& pills)
{
// place pills at its new position in grid
void placeItem(char g[][SIZEX], const Item& item);
for (size_t i(0); i < pills.size(); ++i)
placeItem(grid, pills.at(i));
}
//---------------------------------------------------------------------------
//----- move items on the grid
//---------------------------------------------------------------------------
void updateGameData(char g[][SIZEX], Item& spot, int key, string& mess, int& pillsLeft, int zombiesLeft, int& Lives, vector<Item>& pills)
{
//move spot in required direction
void placeItem(char g[][SIZEX], const Item& item);
bool isArrowKey(const int k);
void setKeyDirection(int k, int& dx, int& dy);
void Death(const char g[][SIZEX], Item& spot, int& Lives, int& key);
mess = " "; //reset message to blank
//calculate direction of movement for given key
int dx(0), dy(0);
setKeyDirection(key, dx, dy);
//check new target position in grid and update game data (incl. spot coordinates) if move is possible
switch (g[spot.y + dy][spot.x + dx])
{
//...depending on what's on the target position in grid...
case HOLE: // can move onto
Lives--; // loose a life
if (Lives <= 0)
{
Death(g, spot, Lives, key);
}
spot.x += dx;
spot.y += dy;
break;
case TUNNEL: //can move
spot.y += dy;
spot.x += dx;
break;
case WALL: //can't move that way
mess = "CANNOT GO THERE!";
break;
case PILL: // can move
spot.y += dy;
spot.x += dx;
Lives++; // give an extra life
pillsLeft--; // update the pill count
for (size_t i(0); i < pills.size(); ++i) // check which pill is being eaten out of every pill
{
if (spot.y == pills.at(i).y && spot.x == pills.at(i).x)
{
pills.at(i).symbol = ' '; // get rid of the pill
pills.at(i).x = 55;
pills.at(i).y = 55;
}
}
break;
case ZOMBIE: // take a life
Death(g, spot, Lives, key); // take a life and respawn player
break;
}
}
//---------------------------------------------------------------------------
//----- process key
//---------------------------------------------------------------------------
void setKeyDirection(const int key, int& dx, int& dy)
{
//calculate direction indicated by key
bool isArrowKey(const int k);
switch (key) //...depending on the selected key...
{
case LEFT: //when LEFT arrow pressed...
dx = -1; //decrease the X coordinate
dy = 0;
break;
case RIGHT: //When RIGHT arrow pressed...
dx = +1; //Increase the X coordinate
dy = 0;
break;
case UP: //When UP arrow is pressed...
dx = 0;
dy = -1; //Decrease the y coordinate
break;
case DOWN: //When DOWN arrow is pressed.
dx = 0;
dy = +1; //Increase the y coordinate
}
}
int getKeyPress()
{
//get key or command (in uppercase) selected by user
int keyPressed;
keyPressed = _getch(); //read in the selected arrow key or command letter
while (keyPressed == 224) //ignore symbol following cursor key
keyPressed = _getch();
return keyPressed; //return it in uppercase
}
bool isArrowKey(const int key)
{
//check if the key pressed is an arrow key (also accept 'K', 'M', 'H' and 'P')
return (key == LEFT) || (key == RIGHT) || (key == UP) || (key == DOWN);
}
bool isCheatKey(const int key)
{
// check if the key pressed is a cheat key (E, F, X)
return (key == EAT) || (key == FREEZE) || (key == EXTERMINATE);
}
bool wantsToQuit(const int key)
{
//check if the user wants to quit (when key is 'Q' or 'q')
return key == QUIT;
}
void usedCheatKey(const int key, vector<Item>& pills, int MAXPILLS, int& pillsLeft, int zombiesLeft, bool& exterminate, bool& freezeActive, int& Lives)
{
Legit = false; // toggel so the score won't be recoreded
switch (key) // depenent on the key...
{
case EAT: // destory all pills and add health
Lives += pillsLeft;
pillsLeft = 0;
for (size_t i(0); i < pills.size(); ++i)
{
pills.at(i).symbol = ' ';
}
break;
case EXTERMINATE: // toggle kill all zombies or respawn all zombies
exterminate = !exterminate;
break;
case FREEZE: // toggle freeze all zombie locations
freezeActive = !freezeActive;
break;
}
}
//---------------------------------------------------------------------------
//----- display info on screen
//---------------------------------------------------------------------------
string tostring(int x)
{
//convert an integer to a string
std::ostringstream os;
os << x;
return os.str();
}
string tostring(char x)
{
//convert a char to a string
std::ostringstream os;
os << x;
return os.str();
}
void showMessage(const WORD backColour, const WORD textColour, int x, int y, const string message)
{
//display a string using specified colour at a given position
Gotoxy(x, y);
SelectBackColour(backColour);
SelectTextColour(textColour);
cout << message;
}
void paintEntryScreen(bool canPaint, string& name)
{
//canPaint = false;
//display time and date
SelectBackColour(clGreen);
showMessage(clDarkGrey, clYellow, 40, 1, "FoP Task 1c: April 2018");
showMessage(clDarkGrey, clYellow, 40, 2, "DATE: " + GetDate());
showMessage(clDarkGrey, clYellow, 40, 3, "Time: " + GetTime());
//display menu options available
showMessage(clDarkGrey, clYellow, 40, 5, "TO MOVE USE KEYBOARD ARROWS ");
showMessage(clDarkGrey, clYellow, 40, 6, "TO FREEZE ZOMBIES PRESS 'F' ");
showMessage(clDarkGrey, clYellow, 40, 7, "TO KILL ZOMBIES PRESS 'X' ");
showMessage(clDarkGrey, clYellow, 40, 8, "TO EAT ALL PILLS PRESS 'E' ");
showMessage(clDarkGrey, clYellow, 40, 9, "TO QUIT ENTER 'Q' ");
//Show game title
showMessage(clDarkGrey, clYellow, 10, 5, "--------------------");
showMessage(clDarkGrey, clYellow, 10, 6, "| SPOT AND ZOMBIES |");
showMessage(clDarkGrey, clYellow, 10, 7, "--------------------");
//Group number and names
showMessage(clDarkGrey, clYellow, 10, 14, "Group CS4G2c - 2017-18 ");
showMessage(clDarkGrey, clYellow, 10, 15, "Tom Hoare \t 26037108");
showMessage(clDarkGrey, clYellow, 10, 16, "Daniel Lomax \t 27020576");
showMessage(clDarkGrey, clYellow, 10, 17, "Jack Parkin \t 27022282");
//Take name input
showMessage(clDarkGrey, clYellow, 10, 20, "Enter your name: ");
cin >> name;
system("cls");
}
void paintGame(const char g[][SIZEX], string mess, int& Lives, int& pillsLeft, int zombiesLeft, string& name)
{
//display game title, messages, maze, spot and other items on screen
string tostring(int x);
string tostring(char x);
void showMessage(const WORD backColour, const WORD textColour, int x, int y, const string message);
void paintGrid(const char g[][SIZEX]);
// title
showMessage(clBlack, clYellow, 0, 0, "___GAME___");
// time and date
showMessage(clDarkGrey, clYellow, 40, 1, "FoP Task 1c: April 2018");
showMessage(clDarkGrey, clYellow, 40, 2, "DATE: " + GetDate());
showMessage(clDarkGrey, clYellow, 40, 3, "Time: " + GetTime());
//display menu options available
showMessage(clDarkGrey, clYellow, 40, 5, "TO MOVE USE KEYBOARD ARROWS ");
showMessage(clDarkGrey, clYellow, 40, 6, "TO FREEZE ZOMBIES PRESS 'F' ");
showMessage(clDarkGrey, clYellow, 40, 7, "TO KILL ZOMBIES PRESS 'X' ");
showMessage(clDarkGrey, clYellow, 40, 8, "TO QUIT ENTER 'Q' ");
//Display stats
showMessage(clDarkGrey, clYellow, 40, 10, "REMAINING LIVES: " + tostring(Lives));
showMessage(clDarkGrey, clYellow, 40, 11, "ZOMBIES LEFT: " + tostring(zombiesLeft));
showMessage(clDarkGrey, clYellow, 40, 12, "PILLS LEFT: " + tostring(pillsLeft));
// display players entered name
showMessage(clDarkGrey, clYellow, 40, 15, "PLAYER'S NAME: " + name);
//print auxiliary messages if any
showMessage(clBlack, clWhite, 40, 18, mess); //display current message
//display grid contents
paintGrid(g);
}
void paintGrid(const char g[][SIZEX])
{
//display grid content on screen
SelectBackColour(clBlack);
SelectTextColour(clWhite);
Gotoxy(0, 2);
for (int row(0); row < SIZEY; ++row)
{
for (int col(0); col < SIZEX; ++col)
cout << g[row][col]; //output cell content
cout << endl;
}
}
void ScoreIncrease(int Score)
{
if (Legit) // as long as the player hasn't used a cheat, the score can be increased
{
Score++;
}
}
void Death(const char g[][SIZEX], Item& spot, int& Lives, int& key)
{
void endProgram(int& key);
Lives--;
if (Lives > 0) // aslong as the player has lives left, set the players position randomly(respawn)
{
int yPos = Random(SIZEY - 2);
int xPos = Random(SIZEX - 2);
while (g[yPos][xPos] != TUNNEL)
{
yPos = Random(SIZEY - 2);
xPos = Random(SIZEX - 2);
}
spot.y = yPos;
spot.x = xPos;
}
else // if the player has run out of lives, end the program
{
endProgram(key);
}
}
void endProgram(int& key)
{
key = 'Q';
void showMessage(const WORD backColour, const WORD textColour, int x, int y, const string message);
showMessage(clRed, clYellow, 40, 8, "");
system("pause"); //hold output screen until a keyboard key is hit
}
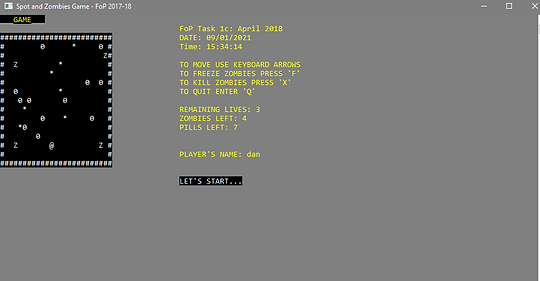