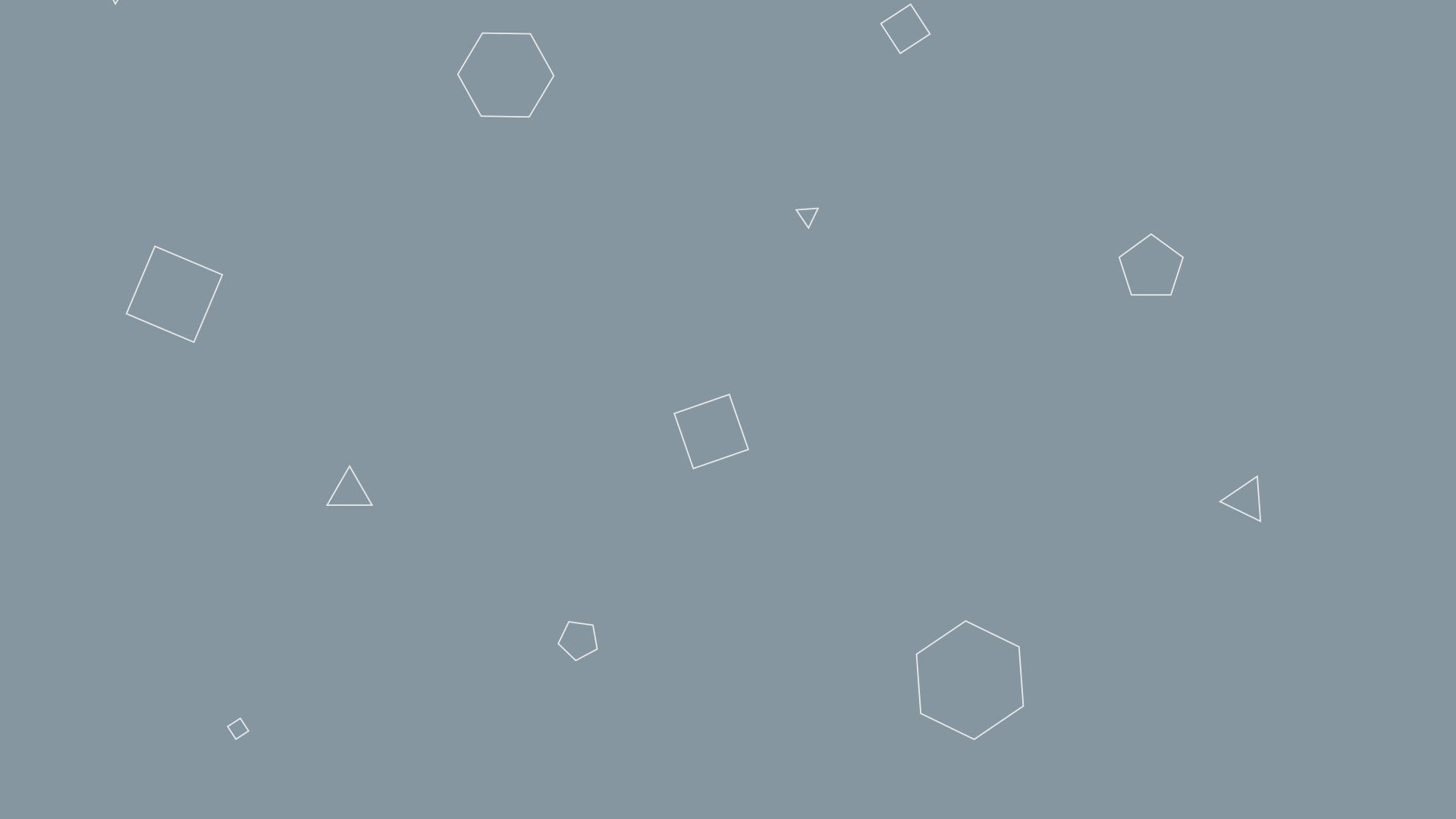
ASM/C++ Fundamentals of computer architecture assignment - encrypt and decrypt
char EKey = 'j';
#define StudentName "Daniel Lynham"
#define MAXCHARS 6 // feel free to alter this, but 6 is the minimum
using namespace std;
#include <conio.h> // for kbhit
#include <windows.h>
#include <string> // for strings
#include <fstream> // file I/O
#include <iostream> // for cin >> and cout <<
#include <iomanip> // for fancy output
#include "TimeUtils.h" // for GetTime, GetDate, etc.
#define dollarchar '$' // string terminator
char OChars[MAXCHARS],
EChars[MAXCHARS],
DChars[MAXCHARS]; // Global Original, Encrypted, Decrypted character strings
//----------------------------- C++ Functions ----------------------------------------------------------
void get_char (char& a_character)
{
cin >> a_character;
while (((a_character < '0') | (a_character > 'z')) && (a_character != dollarchar))
{ cout << "Alphanumeric characters only, please try again > ";
cin >> a_character;
}
}
//-------------------------------------------------------------------------------------------------------------
void get_original_chars (int& length)
{ char next_char = ' ';
length = 0;
get_char (next_char);
while ((length < MAXCHARS) && (next_char != dollarchar))
{
OChars [length++] = next_char;
get_char (next_char);
}
}
//---------------------------------------------------------------------------------------------------------------
//----------------- ENCRYPTION ROUTINES -------------------------------------------------------------------------
void encrypt_chars(int length, char EKey)
{
char temp_char;
for (int i = 0; i < length; i++)
{
temp_char = OChars[i]; // Get next char
__asm {
push eax // save eax current value onto the stack
push ecx // save ecx current value onto the stack
movzx ecx, temp_char // use ecx to encrpyt unsigned temp_char
lea eax, EKey // load adress of EKey. Each char will have its own EKey
push eax // put EKey's adress onto stack ([ebp + 12])
push ecx // put temp_char on stack([ebp + 8])
call encrypt_8 // encrpyt current temp_char
add esp, 8 // clear stack of data used t encrypt previous char
mov temp_char, al // store encrypted char to be put into new array
pop ecx // restore eax current value from the stack
pop eax // restore ecx current value from the stack
}
EChars[i] = temp_char; // save encrpyted char
}
return;
__asm
{
encrypt_8:
push ebp // save back pointer onto stack
mov ebp, esp // save stack position in ebp - use ebp as parameter pointer
mov ecx, [ebp + 8] // get EKey's address from stack so it's ready for use
mov eax, [ebp + 12] // get temp_char from stack so it's ready for use
push ecx // save encrypted char
mov edi, eax // move Ekey address into EDI
movzx eax, byte ptr[eax] // get Ekey's actual value
and eax, 0x3f // restrain the size of the Ekey to 3F
rol al, 1 // alter EKey -- move EKey's MSB to its LSB
mov byte ptr[edi], al // store updated EKey's address to EDI
cmp eax, 0x00 // test if Ekey is 0. We want a char
jnz x8 // move on with encryption
mov eax, 0x09 // if 0, Ekey becomes 9 to become useable
x8: mov edx, eax // store EKey
pop eax // get temp_char for encryption
y8: dec eax // ** create a loop
dec edx // ** temp_char value - EKey value
jnz y8 // ** = encrypted char
rol al, 1 // randomise temp_char -- move temp_char's MSB to its LSB
not al // randomise temp_char -- NOT the binary value of temp_char
pop ebp // get position in EBP back
ret
}
}
//*** end of encrypt_chars function
//---------------------------------------------------------------------------------------------------------------
//---------------------------------------------------------------------------------------------------------------
//----------------- DECRYPTION ROUTINES -------------------------------------------------------------------------
//
void decrypt_chars (int length, char EKey)
{
/*** to be written by you ***/
char temp_char;
for (int i = 0; i < length; i++)
{
temp_char = EChars[i]; // Get next char
__asm {
push eax // save eax current value onto the stack
push ecx // save ecx current value onto the stack
movzx ecx, temp_char // use ecx to encrpyt unsigned temp_char
lea eax, EKey // load adress of EKey. Each char will have its own EKey
push eax // put EKey's adress onto stack ([ebp + 12])
push ecx // put temp_char on stack([ebp + 8])
call decrypt_8 // encrpyt current temp_char
add esp, 8 // clear stack of data used t encrypt previous char
mov temp_char, al // store encrypted char to be put into new array
pop ecx // restore eax current value from the stack
pop eax // restore ecx current value from the stack
}
DChars[i] = temp_char; // save encrpyted char
}
return;
__asm
{
decrypt_8:
push ebp // save back pointer onto stack
mov ebp, esp // save stack position in ebp - use ebp as parameter pointer
mov ecx, [ebp + 8] // get EKey's address from stack so it's ready for use
mov eax, [ebp + 12] // get temp_char from stack so it's ready for use
push ecx // save encrypted char
mov edi, eax // move Ekey address into EDI
movzx eax, byte ptr[eax] // get Ekey's actual value
and eax, 0x3f // restrain the size of the Ekey to 3F
rol al, 1 // alter EKey -- move EKey's MSB to its LSB
mov byte ptr[edi], al // store updated EKey's address to EDI
cmp eax, 0x00 // test if Ekey is 0. We want a char
jnz x8 // move on with encryption
mov eax, 0x09 // if 0, Ekey becomes 9 to become useable
x8: mov edx, eax // store EKey
pop eax // get temp_char for encryption
not al
ror al, 1
y8: dec eax
inc edx
jnz y8
//y8 : dec eax // ** create a loop
//dec edx // ** temp_char value - EKey value
//jnz y8 // ** = encrypted char
//rol al, 1 // randomise temp_char -- move temp_char's MSB to its LSB
//not al // randomise temp_char -- NOT the binary value of temp_char
pop ebp // get position in EBP back
ret
}
}
//*** end of decrypt_chars function
//---------------------------------------------------------------------------------------------------------------
int main(void)
{
int char_count (0); // The number of actual characters entered (upto MAXCHARS limit).
cout << "\nPlease enter upto " << MAXCHARS << " alphanumeric characters: ";
get_original_chars (char_count);
ofstream EDump;
EDump.open("EncryptDump.txt", ios::app);
EDump << "\n\nFoCA Encryption program results (" << StudentName << ") Encryption key = '" << EKey << "'";
EDump << "\nDate: " << GetDate() << " Time: " << GetTime();
// Display and save initial string
cout << "\n\nOriginal string = " << OChars << "\tHex = ";
EDump<< "\n\nOriginal string = " << OChars << "\tHex = ";
for (int i = 0; i < char_count; i++)
{
cout << hex << setw(2) << setfill('0') << ((int(OChars[i])) & 0xFF) << " ";
EDump<< hex << setw(2) << setfill('0') << ((int(OChars[i])) & 0xFF) << " ";
};
//*****************************************************
// Encrypt the string and display/save the result
encrypt_chars (char_count, EKey);
cout << "\n\nEncrypted string = " << EChars << "\tHex = ";
EDump<< "\n\nEncrypted string = " << EChars << "\tHex = ";
for (int i = 0; i < char_count; i++)
{
cout << ((int(EChars[i])) & 0xFF) << " ";
EDump<< ((int(EChars[i])) & 0xFF) << " ";
}
//*****************************************************
// Decrypt the encrypted string and display/save the result
decrypt_chars (char_count, EKey);
cout << "\n\nDecrypted string = " << DChars << "\tHex = ";
EDump<< "\n\nDecrypted string = " << DChars << "\tHex = ";
for (int i = 0; i < char_count; i++)
{
cout << ((int(DChars[i])) & 0xFF) << " ";
EDump<< ((int(DChars[i])) & 0xFF) << " ";
}
//*****************************************************
cout << "\n\n\n";
EDump << "\n\n-------------------------------------------------------------";
EDump.close();
system("PAUSE");
return (0);
} // end of whole encryption/decryption program --------------------------------------------------------------------
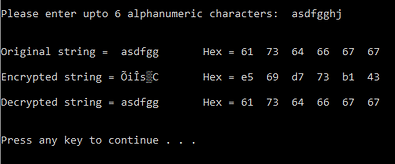